Private Function GetErrorText(ByVal ex As Exception) As String Dim err As String = ex.Message If ex.InnerException IsNot Nothing Then err &= ' - More details: ' & ex.InnerException.Message End If Return err End Function Then you can just do MessageBox.Show(GetErrorText(ex)). An object that describes the error that caused the current exception. The InnerException property returns the same value as was passed into the Exception (String, Exception) constructor, or null if the inner exception value was not supplied to the constructor. This property is read-only.
- Vb.net Ex.innerexception
- Vb.net Innerexception Null
- Vb.net Throw New Exception Innerexception
- Vb.net Ex.innerexception.message
- Vb.net Innerexception 使い方
- Vb.net Check Innerexception
- VB.Net Basic Tutorial
- VB.Net Advanced Tutorial
Vb.net Ex.innerexception

- VB.Net Useful Resources
- Selected Reading
Following table shows all the logical operators supported by VB.Net. Assume variable A holds Boolean value True and variable B holds Boolean value False, then −
Vb.net Innerexception Null
Operator | Description | Example |
---|---|---|
And | It is the logical as well as bitwise AND operator. If both the operands are true, then condition becomes true. This operator does not perform short-circuiting, i.e., it evaluates both the expressions. | (A And B) is False. |
Or | It is the logical as well as bitwise OR operator. If any of the two operands is true, then condition becomes true. This operator does not perform short-circuiting, i.e., it evaluates both the expressions. | (A Or B) is True. |
Not | It is the logical as well as bitwise NOT operator. Used to reverse the logical state of its operand. If a condition is true, then Logical NOT operator will make false. | Not(A And B) is True. |
Xor | It is the logical as well as bitwise Logical Exclusive OR operator. It returns False if both expressions are True or both expressions are False; otherwise, it returns True. This operator does not perform short-circuiting, it always evaluates both expressions and there is no short-circuiting counterpart of this operator | A Xor B is True. |
AndAlso | It is the logical AND operator. It works only on Boolean data. It performs short-circuiting. | (A AndAlso B) is False. |
OrElse | It is the logical OR operator. It works only on Boolean data. It performs short-circuiting. | (A OrElse B) is True. |
IsFalse | It determines whether an expression is False. | |
IsTrue | It determines whether an expression is True. |
Try the following example to understand all the logical/bitwise operators available in VB.Net −
When the above code is compiled and executed, it produces the following result −

Vb.net Throw New Exception Innerexception
Back to: C#.NET Tutorials For Beginners and Professionals
Inner Exception in C# with an Example
In this article, I am going to discuss the Inner Exception in C# with examples. Please read our previous article before proceeding to this article where we discussed how to create a Custom Exception in C# with examples. At the end of this article, you will understand what is Inner Exception and its need in C#.
What is Inner Exception in C#?The Inner Exception in C# is a property of an exception class. When there is a series of exceptions, then the most current exception obtains the previous exception details in the InnerException property. In order words, we can say that the InnerException property returns the original exception that caused the current exception. If this is not clear at the moment, then don't worry we will discuss this with examples.
Inner Exception Example in C#:Let us say we have an exception inside a try block which is throwing DivideByZeroExceptionand the catch block catches that exception and then tries to write that exception it to a file. However, if the file path is not found, then the catch block is also going to throw FileNotFoundException.
Let's say the outside try block catches this FileNotFoundException exception, but how about the actual DivideByZeroExceptionthat was thrown? Is it lost? No, the InnerException property of the Exception class contains the actual exception.
Vb.net Ex.innerexception.message
Example:Let us understand the Inner Exception with an example. In order to see the inner exception, we have to make this program cause an exception to fail. To do that we have 3 options
Vb.net Innerexception 使い方
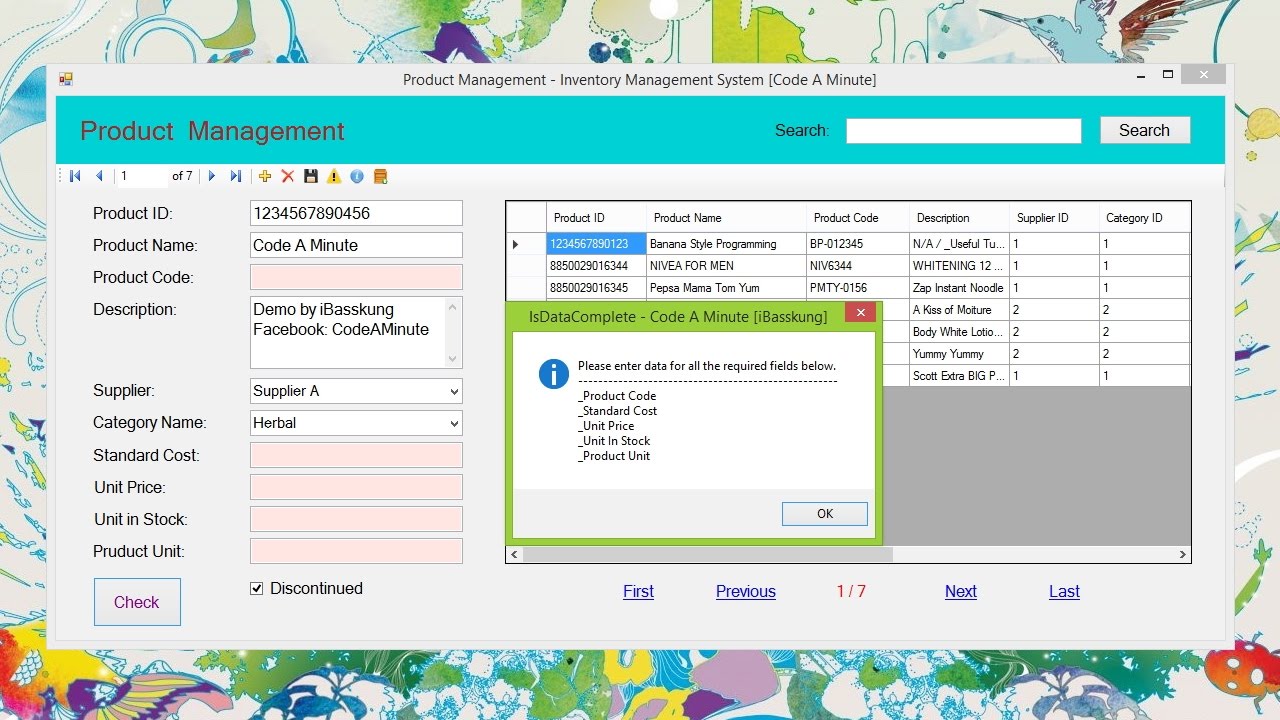
- VB.Net Advanced Tutorial
Vb.net Ex.innerexception
- VB.Net Useful Resources
- Selected Reading
Following table shows all the logical operators supported by VB.Net. Assume variable A holds Boolean value True and variable B holds Boolean value False, then −
Vb.net Innerexception Null
Operator | Description | Example |
---|---|---|
And | It is the logical as well as bitwise AND operator. If both the operands are true, then condition becomes true. This operator does not perform short-circuiting, i.e., it evaluates both the expressions. | (A And B) is False. |
Or | It is the logical as well as bitwise OR operator. If any of the two operands is true, then condition becomes true. This operator does not perform short-circuiting, i.e., it evaluates both the expressions. | (A Or B) is True. |
Not | It is the logical as well as bitwise NOT operator. Used to reverse the logical state of its operand. If a condition is true, then Logical NOT operator will make false. | Not(A And B) is True. |
Xor | It is the logical as well as bitwise Logical Exclusive OR operator. It returns False if both expressions are True or both expressions are False; otherwise, it returns True. This operator does not perform short-circuiting, it always evaluates both expressions and there is no short-circuiting counterpart of this operator | A Xor B is True. |
AndAlso | It is the logical AND operator. It works only on Boolean data. It performs short-circuiting. | (A AndAlso B) is False. |
OrElse | It is the logical OR operator. It works only on Boolean data. It performs short-circuiting. | (A OrElse B) is True. |
IsFalse | It determines whether an expression is False. | |
IsTrue | It determines whether an expression is True. |
Try the following example to understand all the logical/bitwise operators available in VB.Net −
When the above code is compiled and executed, it produces the following result −
Vb.net Throw New Exception Innerexception
Back to: C#.NET Tutorials For Beginners and Professionals
Inner Exception in C# with an Example
In this article, I am going to discuss the Inner Exception in C# with examples. Please read our previous article before proceeding to this article where we discussed how to create a Custom Exception in C# with examples. At the end of this article, you will understand what is Inner Exception and its need in C#.
What is Inner Exception in C#?The Inner Exception in C# is a property of an exception class. When there is a series of exceptions, then the most current exception obtains the previous exception details in the InnerException property. In order words, we can say that the InnerException property returns the original exception that caused the current exception. If this is not clear at the moment, then don't worry we will discuss this with examples.
Inner Exception Example in C#:Let us say we have an exception inside a try block which is throwing DivideByZeroExceptionand the catch block catches that exception and then tries to write that exception it to a file. However, if the file path is not found, then the catch block is also going to throw FileNotFoundException.
Let's say the outside try block catches this FileNotFoundException exception, but how about the actual DivideByZeroExceptionthat was thrown? Is it lost? No, the InnerException property of the Exception class contains the actual exception.
Vb.net Ex.innerexception.message
Example:Let us understand the Inner Exception with an example. In order to see the inner exception, we have to make this program cause an exception to fail. To do that we have 3 options
Vb.net Innerexception 使い方
Vb.net Check Innerexception
When you run the above program it will give us the following output.
In the next article, I am going to discuss theException Handling Abusein C#. Here, in this article, I try to explain the Inner Exception in C# with examples. I would like to have your feedback. Please post your feedback, question, or comments about this article.